blog
5 Best Python Classes and Objects exercises for Beginners
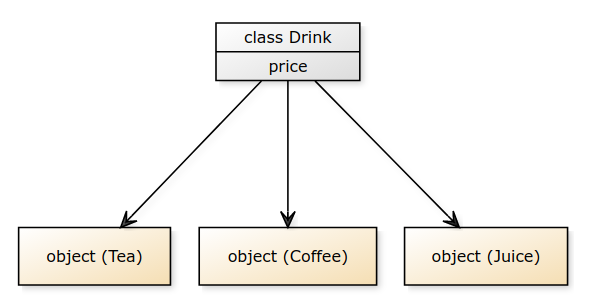
These Python classes and objects exercises for beginners will help you practice working with Python classes and objects. They cover various aspects of object-oriented programming, including creating classes, inheritance, encapsulation, and using Dunder methods. You can progressively work through these exercises to build a strong foundation in working with classes and objects in Python.
Python classes and objects exercises for beginners
See Also:
- How to find a np transpose matrix in Python
- Numpy Transpose Matrix in Python:
- Python Classes and Objects Exercises for Beginners
- Functional Programming vs OOP: Best Comparison
- Python Inheritance Constructor | Super | Override | Init
- Using Python Lamda if else | elif | function | syntex
- How to Convert Python JSON to CSV using Python Libraries
- What does Numpy Arange do | np.arange
- How Many Data Types in Python with examples
- Assignment Operator in Python: Variables and Values
- What is Python Numpy linspace integer?
- How to Add Numpy Append Array
- np where multiple conditions and values
- np zeros Python | What does 0 mean in Python
- Unveiling the Distinctions: Web Crawler vs Web Scrapers
- How to Make Money with Web Scraping Using Python
- How to Type Cast in Python with the Best 5 Examples
- Best Variable Arguments in Python
- 5 Best AI Prompt Engineering Certifications Free
- 5 Beginner Tips for Solving Python Coding Challenges
- Exploring Python Web Development Example Code
- “Python Coding Challenges: Exercises for Success”
- ChatGPT Prompt Engineering for Developers:
- How to Make AI-Generated Video|AI video generator
- 12 Best Python Web Scraping Tools and Libraries with Pros & Cons
- 7 Best Python Web Scraping Library To Master Data Extraction
- Explore the World of Character AI Generator
- Boolean Operators in Python Examples (And|Or|Not)
- Python List Slicing: 3 Best Advanced Techniques
- Roadmap: Navigating Your Career with a Masters in Data Analytics: