Using Python Lamda if else | elif | function | syntex with example
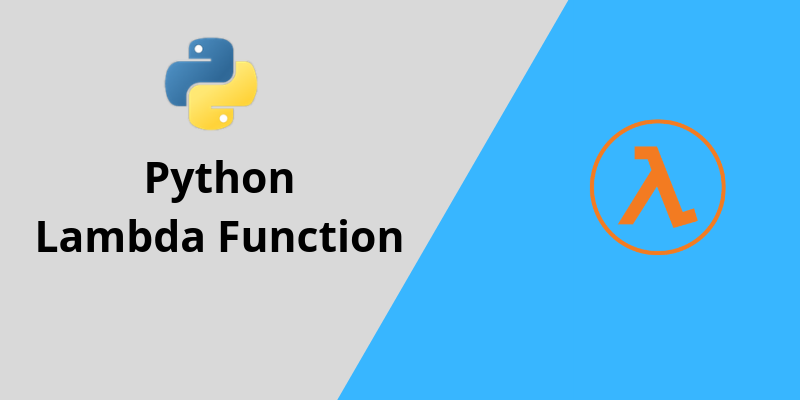
Python Lamda if, the function is an expression to create small, anonymous functions for simple conditional operations. It has the following format:
lambda arguments: value_if_true if condition else value_if_false.
Generally little anonymous function is called a lambda function. Although a lambda function can only have one expression, it can have an unlimited number of parameters.
Introduction: Python Lambda
A Python lambda function, often referred to simply as a “lambda,” is a small, anonymous function that can be used for concise and one-time operations. Lambdas are typically limited to a single expression and are used when you don’t need a full function definition.
Python Syntax:
lambda arguments : expression
After the expression is run, the following outcome is returned:
Example:
Add 20 to the argument a
, and return the result:
y = lambda a : a + 20
print(y(5)) #output will be 25
Any number of arguments can be passed to lambda functions:
Example:
Multiply argument x with argument y and return the result:
x = lambda a, b : a * b
print(x(5, 6)) #output will be 30
Why Use Lambda Functions?
When lambdas are used as anonymous functions inside other functions, their power is more clearly demonstrated.
Let’s say you have a function definition that accepts a single parameter that will undergo an arbitrary number of multiplicities:
Example:
Create a function that always doubles the input number by using that function definition:
def myfunc(n):
return lambda a : a * nmydoubler = myfunc(2)print(mydoubler(11))
Lambda functions, also known as anonymous functions or lambda expressions, are a feature in many programming languages, including Python, that allows you to create small, one-time-use functions without formally defining them using the def
keyword. They are named after the lambda calculus, a mathematical notation for expressing functions. Lambda functions have several use cases and benefits:
- Conciseness: Lambda functions are a compact way to define simple functions, especially when you need a quick, short function for a specific task. They can make your code more concise and easier to read, particularly when used with functions that accept other functions as arguments (e.g.,
map
,filter
,sorted
). - Readability: Lambda functions can make your code more readable when the function’s logic is simple and doesn’t require a formal function definition. This can reduce the cognitive load for someone reading your code, as they don’t need to jump between function definitions.
- No Need for Function Names: With lambda functions, you don’t need to assign a name to the function. This is useful when you want to define a function on the fly without polluting the namespace with unnecessary function names.
- Functional Programming: Lambda functions are often used in functional programming, where they can be passed as arguments to other functions. For example, you can use
map
,filter
, andreduce
functions with lambda functions to perform operations on collections of data. - Closures: Lambda functions can capture variables from their surrounding scope, creating closures. This is useful when you need to create functions with context-specific behavior, such as when defining custom sorting or filtering criteria.
- Convenience: In some cases, using a lambda function can be more convenient than defining a separate function when you need a simple, short-lived function for a specific task.
Here’s an example in Python:
# Using a lambda function to sort a list of tuples by the second element
data = [(1, 5), (3, 2), (2, 8)]
sorted_data = sorted(data, key=lambda x: x[1])
In this example, the lambda function lambda x: x[1]
is used to specify the sorting key. It makes the code more concise and avoids the need to define a separate function for this one-time use case.
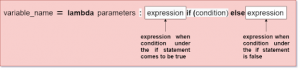
Using the Python Lamda if function:
In Python, a lambda function with an if
statement is used to create concise conditional expressions. It allows you to define a simple, one-line function that returns one value if a condition is true and another value if it’s false. For example, you can use lambda x: "Even" if x % 2 == 0 else "Odd"
it to determine if a number is even or odd. It’s a compact way to express conditional logic without the need for a full function definition.
It has the following format:
lambda arguments: value_if_true if condition else value_if_false
Here’s a breakdown:
arguments
These are the input parameters to the lambda function.value_if_true
: The value or expression to be returned if the condition is true.condition
The condition that, when true, results invalue_if_true
being returned.value_if_false
The value or expression to be returned if the condition is false.
For example, you can use a lambda function like this:
is_even = lambda x: “Even” if x % 2 == 0 else “Odd”
In this case, is_even
is a lambda function that takes a single argumentx
. It checks if x
is even by using the conditionx % 2 == 0
. If the condition is true, it returns “Even”; otherwise, it returns “Odd.”
Python Lambda if functions are handy for simple, on-the-fly conditional operations and are often used when you need a small function for tasks like mapping or filtering elements in a collection.
Using the Python lambda if elif function
In Python, lambda functions withif-elif
conditions can be used to create small, anonymous functions that perform different actions based on different conditions. Here’s the basic structure:
lambda arguments: value_if_condition1 if condition1 else value_if_condition2 if condition2 else value_if_condition3
Here’s a breakdown:
arguments
These are the input parameters to the lambda function.condition1
,condition2
,condition3
, etc.: These are the conditions you want to test in order. When a condition is true, the corresponding value is returned.value_if_condition1
,value_if_condition2
,value_if_condition3
, etc.: These are the values or expressions to be returned if the corresponding condition is true.
Here’s an example:
classify_grade = lambda score: “A” if score >= 90 else “B” if score >= 80 else “C” if score >= 70 else “D” if score >= 60 else “F”
In this case, classify_grade
is a lambda function that takes a single argumentscore
. It assigns a grade based on the value of score
using a series of if-elif
conditions. Depending on the score, it returns “A,” “B,” “C,” “D,” or “F.”
Python lambdaif-elif
conditions are useful for creating concise, one-line functions that perform conditional operations. However, they should be used judiciously for simple tasks, as overly complex lambda functions can become difficult to read and maintain.
Using the Python lambda if else function
In Python, a lambda function with an if-else
expression is a compact way to create small, anonymous functions that perform conditional operations. Here’s the basic structure:
lambda arguments: value_if_true if condition else value_if_false
Here’s a breakdown:
arguments
These are the input parameters to the lambda function.value_if_true
The value or expression is to be returned if the condition is true.condition
The condition that, when true, results invalue_if_true
being returned.value_if_false
: The value or expression to be returned if the condition is false.
Here’s an example:
is_even = lambda x: “Even” if x % 2 == 0 else “Odd”
In this case, is_even
is a lambda function that takes a single argumentx
. It checks if x
is even using the condition x % 2 == 0
. If the condition is true, it returns “Even”; otherwise, it returns “Odd.”
Python lambdaif-else
expressions are useful for creating concise, one-line functions for simple conditional operations. They are commonly used with functions like map
, filter
, and reduce
where you need to apply a condition to each element in an iterable. However, they are best suited for relatively simple logic, as overly complex lambda functions can become difficult to read and understand.
Using the Python lambda if else elif function
You can use Python lambda functions with if-else
or elif
statements to create small, anonymous functions that perform conditional operations. Here are some examples:
Lambda with if-else
statement:
greater = lambda x, y: x if x > y else y
result = greater(3, 5) # result will be 5
In this example, the lambda function checks if x
is greater than y
. If true, it returns x
; otherwise, it returns y
.
Lambda with if-elif-else
statements:
grade = lambda score: “A” if score >= 90 else “B” if score >= 80 else “C” if score >= 70 else “D” if score >= 60 else “F”
result = grade(85) # result will be “B”
Here, the lambda function assigns a grade based on the value of score
using a series of if-elif-else
conditions.
These Python lambda if-elif-else functions allow you to create concise, one-line functions for conditional operations. However, they are best suited for relatively simple logic. If the logic becomes too complex, it’s often better to use a regular function with a def
statement for better readability and maintainability.
Using Python lambda if or function
In Python, you can use a lambda function in conjunction with the or
operator to create a conditional lambda function. Here’s an example:
check_even_or_odd = lambda x: “Even” if x % 2 == 0 else “Odd”
result1 = check_even_or_odd(4) # result1 will be “Even”
result2 = check_even_or_odd(7) # result2 will be “Odd”
In this Python lambda if or, example, the lambda function check_even_or_odd
takes a single argument x
. It checks if x
is even using the condition x % 2 == 0
. If the condition is true, it returns “Even”; otherwise, it returns “Odd.”
The or
the operator is not used directly in this lambda function. Instead, the lambda function itself includes an if
statement to provide a condition-based result. You can use the or
operator in more complex conditions if needed.
Conclusion:
In conclusion, Python lambda if
statements offer a concise way to create small, anonymous functions for conditional operations. They are defined with a simple structure:
lambda arguments: value_if_true if condition else value_if_false
These lambda functions are useful for performing specific tasks where a full function definition is unnecessary. However, they are best suited for relatively simple conditional logic. When conditions become complex, it’s advisable to use regular functions defined def
for improved readability and maintainability. Lambdas are often used with functions like map
, filter
, and sorted
when applying conditions to elements in an iterable.