Python List Slicing: 3 Best Advanced Techniques
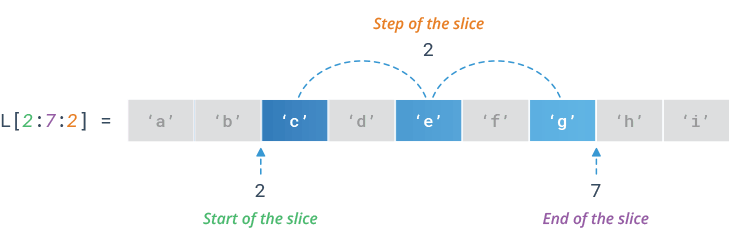
Python List slicing is a powerful and efficient feature that can greatly enhance your ability to work with lists and sequences. By mastering list slicing, you unlock a versatile tool that simplifies many common programming tasks. Python’s list-slicing feature enables us to modify a list element by updating the list at a designated place, as demonstrated in this blog, by providing the list with the values as indicated in the example, the index positions 0 and 1 were updated to black and white, respectively our blog.
Python List Slicing:
Python lists are versatile data structures, and one of the powerful features that make them so flexible is list slicing. List slicing allows you to extract specific portions of a list efficiently. In this guide, we’ll delve into the intricacies of Python list slicing and explore various use cases.
Table of Contents
- Introduction to List Slicing
- What is list slicing?
- Basic syntax:
list[start:stop]
- Understanding the Parameters
- Start index
- Stop index
- Step value
- Basic List Slicing Examples
- Slicing a list from the beginning
- Slicing a list from the end
- Slicing with step values
- Negative Indexing and Reversing a List
- Using negative indices
- Reversing a list by slicing
- Omitting Indices
- Default start, stop, and step values
- Omitting any of the values
- List Slicing with Strings
- Applying list slicing to strings
- Getting substrings efficiently
- Advanced Techniques
- Using list slicing in comprehension
- Modifying elements with slicing
- Creating copies and avoiding pitfalls
- Performance Considerations
- The time complexity of list slicing
- When to avoid list slicing for large lists
- Common Mistakes and Pitfalls
- Off-by-one errors
- Misunderstanding the step value
- In-place modification gotchas
- Real-World Examples
- Extracting data from CSV files
- Parsing log files with list slicing
- Efficient data extraction in data science tasks
- Conclusion
- Recap of key concepts
- Best practices for using Python list slicing
(1) Introduction to List Slicing
-
- What is list slicing?
- Basic syntax:
list[start:stop]
Lists in Python are dynamic and versatile containers that allow you to store and manipulate collections of items. One powerful feature that makes working with lists efficient and expressive is list slicing.
What is List Slicing?
List slicing is the process of extracting a portion of a list, and creating a new list with those elements. It provides a concise and readable way to work with specific subsets of a list, making it a fundamental technique in Python programming.
Basic Syntax: list[start:stop]
The basic syntax for list slicing consists of the list followed by square brackets containing two indices separated by a colon. The start
index indicates where the slice begins, and the stop
index indicates where the slice ends. The resulting slice includes all elements from the start
index up to, but not including, the element at the stop
index.
Here’s a breakdown of the basic syntax:
list
: The name of the list you want to slice.start
: The index from which the slice begins. If omitted, it defaults to the beginning of the list.stop
: The index at which the slice ends. The slice includes all elements up to, but not including, the element at this index. If omitted, it defaults to the end of the list.
Let’s look at a few examples to illustrate the basic syntax:
my_list = [1, 2, 3, 4, 5, 6, 7, 8, 9]
# Slicing from index 2 to 6 (exclusive)
sliced_list = my_list[2:6]print(sliced_list)
# Output: [3, 4, 5, 6]
In this example, the slice begins at index 2 (start
) and ends at index 6 (stop
), creating a new list containing elements [3, 4, 5, 6]
. It’s important to note that the original list remains unchanged.
List slicing becomes even more powerful when you consider additional features, such as step values, negative indices, and omitting indices, which we’ll explore in the subsequent sections of this guide. Stay tuned for a deeper dive into the nuances of list slicing!
(2) Understanding the Parameters in List Slicing:
To delve deeper into Python list slicing, it’s crucial to understand the three parameters: start index, stop index, and step value. These parameters provide fine-grained control over the extraction of elements from a list.
Start Index:
- The
start
index specifies the position in the list where the slice begins. - If the
start
index is omitted, it defaults to the beginning of the list. - A positive integer refers to the position from the start of the list.
- A negative integer refers to the position from the end of the list.
my_list = [1, 2, 3, 4, 5, 6, 7, 8, 9]
# Start index is 2
sliced_list = my_list[2:]
print(sliced_list)
# Output: [3, 4, 5, 6, 7, 8, 9]
Stop Index:
- The
stop
index specifies the position in the list where the slice ends. - The slice includes elements up to, but not including, the element at the
stop
index. - If the
stop
index is omitted, it defaults to the end of the list. - Similar to the
start
index, a positive or negative integer can be used.
my_list = [1, 2, 3, 4, 5, 6, 7, 8, 9]
# Stop index is 6
sliced_list = my_list[:6]
print(sliced_list)
# Output: [1, 2, 3, 4, 5, 6]
Step Value:
- The
step
value determines the interval between elements in the slice. - A positive integer indicates forward movement through the list.
- A negative integer indicates backward movement.
- If the
step
value is omitted, it defaults to 1.
In this example, the slice starts from index 1 and includes every second element, resulting in [2, 4, 6, 8]
.
Understanding these parameters allows you to precisely control which elements are included in your slice, making list slicing a powerful tool for working with data in Python.
(3) Basic List Slicing Examples:
Let’s explore some fundamental Python list slicing examples to understand how to extract specific subsets of a list using Python’s powerful slicing syntax.
1. Slicing a List from the Beginning:
-
- To slice a list from the beginning, you can omit the start index, and Python will assume you want to start from the first element.
my_list = [1, 2, 3, 4, 5, 6, 7, 8, 9]
# Slicing from the beginning (omitting start index)
sliced_list = my_list[:]
print(sliced_list)
# Output: [1, 2, 3, 4, 5, 6, 7, 8, 9]
The colon [:]
indicates that you want to include all elements from the beginning to the end of the list, effectively creating a copy of the original list.
2. Slicing a List from the End:
-
- To slice a list from the end, you can use negative indexing to specify the start index.
my_list = [1, 2, 3, 4, 5, 6, 7, 8, 9]
# Slicing from the end
sliced_list = my_list[-3:]
print(sliced_list)
# Output: [7, 8, 9]
In this example, the slice starts from the third-to-last element (-3
index) and includes all elements until the end of the list.
3. Slicing with Step Values:
-
- The step value controls the spacing between elements in the slice. You can specify a step value by adding a second colon and an integer after the stop index.
my_list = [1, 2, 3, 4, 5, 6, 7, 8, 9]
# Slicing with a step value of 2
sliced_list = my_list[1::2]
print(sliced_list)
# Output: [2, 4, 6, 8]
Here, the slice starts from index 1 and includes every second element, creating a new list with elements [2, 4, 6, 8]
.
(4) Negative Indexing and Reversing a List:
Negative indexing in Python list slicing allows you to access elements from the end of the list by using negative integers as indices. Additionally, you can leverage negative indexing to reverse a list efficiently. Let’s explore both concepts:
1. Using Negative Indices:
-
- Negative indices count from the end of the list, with -1 representing the last element, -2 representing the second-to-last element, and so on.
my_list = [1, 2, 3, 4, 5, 6, 7, 8, 9]
# Using negative indices to slice the last three elements
sliced_list = my_list[-3:]
print(sliced_list)
# Output: [7, 8, 9]
In this example, the slice starts from the third-to-last element (-3
index) and includes all elements until the end of the list.
2. Reversing a List by Slicing:
-
- You can use negative indexing to reverse a list by specifying a step value of -1.
my_list = [1, 2, 3, 4, 5, 6, 7, 8, 9]
# Reversing a list using negative indexing and step value of -1
reversed_list = my_list[::-1]
print(reversed_list)
# Output: [9, 8, 7, 6, 5, 4, 3, 2, 1]
Here, the slice starts from the beginning of the list (::
) and moves through the list in reverse order due to the step value of -1
. This effectively creates a reversed copy of the original list.
Understanding negative indexing and utilizing it to reverse a list is a handy technique, especially when you need to process elements in reverse order. These concepts, when combined with other slicing features, provide powerful tools for manipulating lists in Python.
(5) Omitting Indices in List Slicing:
One of the convenient aspects of Python list slicing is that you can omit indices to use default values, making the syntax more concise and expressive. Let’s explore how you can omit start, stop, or step values.
1. Default Start, Stop, and Step Values:
-
- If you omit the start, stop, or step value in the list slicing syntax, Python will use default values.
- The default start value is the beginning of the list (index 0).
- The default stop value is the end of the list (index equal to the length of the list).
- The default step value is 1.
my_list = [1, 2, 3, 4, 5, 6, 7, 8, 9]
# Using default start, stop, and step values
default_slice = my_list[:]
print(default_slice)
# Output: [1, 2, 3, 4, 5, 6, 7, 8, 9]
In this example, omitting start, stop, and step values creates a slice that includes all elements, effectively making a copy of the original list.
2. Omitting Any of the Values:
-
- You can also omit specific indices while keeping others. For example, if you omit the start index, the slice will begin from the default start (index 0). If you omit the stop index, the slice will extend to the default stop (end of the list).
my_list = [1, 2, 3, 4, 5, 6, 7, 8, 9]
# Omitting the start index
slice_without_start = my_list[:5]
print(slice_without_start)
# Output: [1, 2, 3, 4, 5]# Omitting the stop index
slice_without_stop = my_list[2:]
print(slice_without_stop)
# Output: [3, 4, 5, 6, 7, 8, 9]
In the first example, omitting the start index creates a slice starting from the default start (index 0) up to, but not including, the element at index 5. In the second example, omitting the stop index creates a slice starting from index 2 until the end of the list.
Omitting indices provides a concise way to express common slicing operations without explicitly specifying each index. Understanding the defaults allows you to write more readable and elegant code when working with lists in Python.
(6) Python List Slicing with Strings: Applying Slicing to Strings:
List slicing isn’t exclusive to lists; it’s a versatile technique that can be applied to other sequence types in Python, including strings. Let’s explore how list slicing works with strings and how it can be used to efficiently obtain substrings.
1. Basic String Slicing:
-
- Strings are sequences of characters, and you can use the same list-slicing syntax to obtain substrings from a string.
my_string = “Hello, World!”
# Basic string slicing
sliced_string = my_string[7:12]
print(sliced_string)
# Output: World
In this example, the slice starts from index 7 and includes characters up to, but not including, the element at index 12, resulting in the substring “World.”
2. Getting Substrings Efficiently:
-
- List slicing with strings becomes powerful when combined with other features like step values.
my_string = “Python is amazing!”
# Getting every second character
every_second_char = my_string[::2]
print(every_second_char)
# Output: Pto saaig# Reversing a string
reversed_string = my_string[::-1]
print(reversed_string)
# Output: !gnizama si nohtyP
In the first example, the slice starts from the beginning of the string and includes every second character, resulting in “Pto saaig.” In the second example, the step value of -1 is used to reverse the string efficiently.
3. String Slicing in Action:
-
- String slicing is particularly useful when dealing with text processing tasks, such as extracting specific information or modifying strings.
email = “user@example.com”
# Extracting the username
username = email[:email.index(‘@’)]
print(username)
# Output: user
In this example, string slicing is combined with the index
method to extract the username from an email address efficiently.
String slicing provides an elegant and efficient way to work with substrings in Python. Whether you’re manipulating text data or extracting information from strings, the principles of list slicing can be applied to strings with ease.
(7) Advanced Techniques in List Slicing:
Now, let’s explore some advanced techniques and strategies for using list slicing in Python, including list comprehensions, modifying elements with slicing, and creating copies while avoiding common pitfalls.
1. Using List Slicing in Comprehensions:
-
- List comprehensions provide a concise and expressive way to create lists. You can leverage list slicing within comprehensions to efficiently generate subsets of existing lists.
original_list = [1, 2, 3, 4, 5, 6, 7, 8, 9]
# Using list slicing in a comprehension to get even numbers
even_numbers = [x for x in original_list[1::2]]
print(even_numbers)
# Output: [2, 4, 6, 8]
In this example, list slicing is used within a list comprehension to create a new list containing every second element from the original list.
2. Modifying Elements with Slicing:
-
- List slicing is not only for extracting data; it can also be used to modify elements within a list.
my_list = [1, 2, 3, 4, 5, 6, 7, 8, 9]
# Modifying elements using list slicing
my_list[1:4] = [10, 11, 12]
print(my_list)
# Output: [1, 10, 11, 12, 5, 6, 7, 8, 9]
In this example, the slice my_list[1:4]
is replaced with the new list [10, 11, 12]
, effectively modifying elements in the original list.
3. Creating Copies and Avoiding Pitfalls:
-
- When working with slices, be mindful of creating copies instead of references. Modifying a slice may affect the original list.
Here, original_list[:]
creates a shallow copy, so modifying copied_list
doesn’t affect original_list
. If a deep copy is needed (for nested lists or objects), consider using the copy
module.
These advanced techniques showcase the flexibility and power of list slicing in various scenarios. Whether you’re generating new lists, modifying existing ones, or ensuring data integrity, list slicing provides efficient solutions.
(8) Performance Considerations in List Slicing
While list slicing in Python is a powerful and convenient feature, it’s essential to be aware of its performance characteristics, especially when working with large lists. Let’s discuss the time complexity of list slicing and situations where it might be advisable to avoid slicing for very large lists.
1. Time Complexity of List Slicing:
-
- The time complexity of list slicing is generally O(k), where k is the size of the resulting slice.
- Creating a slice involves copying elements from the original list to a new list.
original_list = [1, 2, 3, 4, 5, 6, 7, 8, 9]
# Creating a slice
sliced_list = original_list[2:6]
In this example, the time complexity of creating sliced_list
is O(4), as it contains four elements.
2. When to Avoid List Slicing for Large Lists:
-
- While list slicing is generally efficient, it’s important to consider its potential performance impact when working with very large lists.
- Creating slices of large lists can consume more memory and time, especially if frequent slicing operations are performed.
large_list = list(range(1, 10**6))
# Creating a slice of a large list
sliced_large_list = large_list[:1000]
In this case, creating sliced_large_list
involves copying the first 1000 elements of large_list
, which might be inefficient for extremely large lists.
3. Alternatives for Large Lists:
-
- For large lists, consider alternatives to list slicing, especially if you need to perform multiple operations.
- Instead of creating slices, iterate over the original list directly, extracting only the necessary elements.
large_list = list(range(1, 10**6))
# Iterating over the original list instead of creating a slice
for element in large_list[:1000]:
# Process each element as needed
pass
This approach avoids the creation of additional lists and can be more memory-efficient.
Remember that the efficiency of list slicing can depend on the specific implementation of Python and the underlying optimizations made by the interpreter. Profiling your code and considering alternative approaches, such as using iterators or generators, can be beneficial when dealing with very large datasets.
(9) Common Mistakes and Pitfalls in List Slicing:
List slicing in Python is a powerful and flexible feature, but there are some common mistakes and pitfalls that developers might encounter. Let’s discuss three of them: off-by-one errors, misunderstanding the step value, and in-place modification gotchas.
1. Off-by-One Errors:
-
- One common mistake is related to off-by-one errors when specifying start and stop indices. Remember that the slice includes elements from the start index up to, but not including, the element at the stop index.
my_list = [1, 2, 3, 4, 5]
# Incorrect: Including the element at index 3
incorrect_slice = my_list[1:4]
# Output: [2, 3, 4]# Correct: Excluding the element at index 3
correct_slice = my_list[1:3]
# Output: [2, 3]
Be cautious when specifying indices to avoid including an undesired extra element in the slice.
2. Misunderstanding the Step Value:
- Another common mistake is misunderstanding the effect of the step value in list slicing. The step value controls the spacing between elements in the slice and can lead to unexpected results if not used correctly.
my_list = [1, 2, 3, 4, 5, 6, 7, 8, 9]
# Incorrect: Using a negative step value without reversing the list
incorrect_slice = my_list[::2]
# Output: [1, 3, 5, 7, 9]# Correct: Reversing the list when using a negative step value
correct_slice = my_list[::-2]
# Output: [9, 7, 5, 3, 1]
When using a negative step value, ensure that it aligns with the direction in which you want to traverse the list.
3. In-Place Modification Gotchas:
-
- Modifying elements within a list slice can lead to unexpected behavior if not handled carefully. Changes made to the slice can affect the original list.
my_list = [1, 2, 3, 4, 5]
# Incorrect: Modifying the slice in-place
my_slice = my_list[1:4]
my_slice[0] = 10
# Output: my_list is now [1, 10, 3, 4, 5]# Correct: Creating a new list with modifications
my_list = [1, 2, 3, 4, 5]
my_slice = my_list[1:4]
my_list[1:4] = [10, 11, 12]
# Output: my_list is now [1, 10, 11, 12, 5]
If in-place modification is not the intended behavior, create a new list with the modifications and assign it back to the original list.
Being aware of these common mistakes and pitfalls will help you use list slicing more effectively and avoid unexpected outcomes in your Python code. Always double.
(10) Real-World Examples of List Slicing:
List slicing is a versatile tool that finds applications in various real-world scenarios. Let’s explore three practical examples: extracting data from CSV files, parsing log files with list slicing, and efficient data extraction in data science tasks.
1. Extracting Data from CSV Files:
-
- CSV (Comma-Separated Values) files are a common format for storing tabular data. List slicing can be used to extract specific columns or rows efficiently.
import csv
# Reading a CSV file
with open(‘data.csv’, ‘r’) as file:
csv_reader = csv.reader(file)
data = list(csv_reader)# Extracting the first column using list slicing
first_column = [row[0] for row in data]
Here, list slicing is employed to create a list (first_column
) containing the values from the first column of the CSV file.
2. Parsing Log Files with List Slicing:
-
- Log files often contain structured data, and list slicing can be useful for extracting relevant information.
# Reading a log file
with open(‘logfile.txt’, ‘r’) as file:
log_data = file.readlines()# Extracting timestamps using list slicing
timestamps = [line[:19] for line in log_data]
In this example, list slicing is used to extract the first 19 characters (timestamp) from each line of a log file.
3. Efficient Data Extraction in Data Science Tasks:
-
- In data science tasks, list slicing can enhance the efficiency of data extraction and manipulation.
import pandas as pd
# Reading a CSV file with pandas
df = pd.read_csv(‘data.csv’)# Extracting a subset of rows using list slicing
subset_df = df[10:20]
Here, list slicing is applied to a DataFrame object in the pandas’ library to extract a subset of rows efficiently.
These real-world examples demonstrate the practical utility of list slicing in tasks ranging from file manipulation to data analysis. Whether you’re dealing with structured data in CSV files, parsing log information, or working with data science tools, list slicing offers a concise and powerful way to handle and extract specific information from sequences in Python.
(11) Recap and Best Practices for Python List Slicing:
In this comprehensive guide, we’ve explored the fundamentals and advanced techniques of Python list slicing. Let’s recap key concepts and highlight best practices for using list slicing effectively in your Python code.
Recap of Key Concepts:
- List Slicing Basics:
- List slicing allows you to extract specific portions of a list using the syntax
list[start:stop:step]
. - The
start
the index indicates where the slice begins, thestop
index indicates where it ends (exclusive), and thestep
value controls the spacing between elements.
- List slicing allows you to extract specific portions of a list using the syntax
- Understanding Parameters:
- The
start
,stop
, andstep
parameters provide fine-grained control over list slicing. - Omitting indices uses default values: beginning for
start
, end forstop
, and 1 forstep
.
- The
- Advanced Techniques:
- List slicing can be used in list comprehensions, enabling the concise creation of new lists.
- Modifying elements with list slicing allows in-place modifications.
- Creating copies using list slicing can be done with care to avoid unexpected behavior.
- Performance Considerations:
- The time complexity of list slicing is generally O(k), where k is the size of the resulting slice.
- Be mindful of potential performance issues, especially with very large lists.
- Common Mistakes and Pitfalls:
- Watch out for off-by-one errors when specifying start and stop indices.
- Understand the impact of the step value, especially when using negative values.
- Be cautious with in-place modifications to avoid unintended side effects.
- Real-World Examples:
- List slicing is applicable in various real-world scenarios, including extracting data from CSV files, parsing log files, and efficient data extraction in data science tasks.
Best Practices for Using Python List Slicing:
- Be Explicit and Readable:
- Clearly specify start, stop, and step values to make your code more readable.
- Use comments to explain complex slicing operations.
- Avoid Unnecessary Copies:
- Be mindful of memory usage, especially when working with large datasets.
- Consider alternatives if you don’t need to create additional lists.
- Test and Debug:
- Test your slicing operations with various inputs to ensure they produce the expected results.
- Use debugging tools to inspect intermediate results during development.
- Consider Alternatives:
- Depending on the task, consider alternatives like iterators, generators, or other data structures.
- Profile Performance:
- Profile your code to identify potential bottlenecks and optimize accordingly.
- Monitor memory usage, especially when dealing with large datasets.
By incorporating these best practices, you can harness the power of Python list slicing effectively and avoid common pitfalls. List slicing is a valuable tool for data manipulation, and mastering it will enhance your ability to work with sequences in Python. Happy coding!
See Also:
- Unveiling the Distinctions: Web Crawler vs Web Scrapers
- How to Make Money with Web Scraping Using Python
- How to Type Cast in Python with the Best 5 Examples
- Best Variable Arguments in Python
- 5 Best AI Prompt Engineering Certifications Free
- 5 Beginner Tips for Solving Python Coding Challenges
- Exploring Python Web Development Example Code
- “Python Coding Challenges: Exercises for Success”
- ChatGPT Prompt Engineering for Developers:
- How to Make AI-Generated Video|AI video generator
- 12 Best Python Web Scraping Tools and Libraries with Pros & Cons
- 7 Best Python Web Scraping Library To Master Data Extraction
- Explore the World of Character AI Generator
- Boolean Operators in Python Examples (And|Or|Not)