How to Convert Python JSON to CSV using Python Libraries
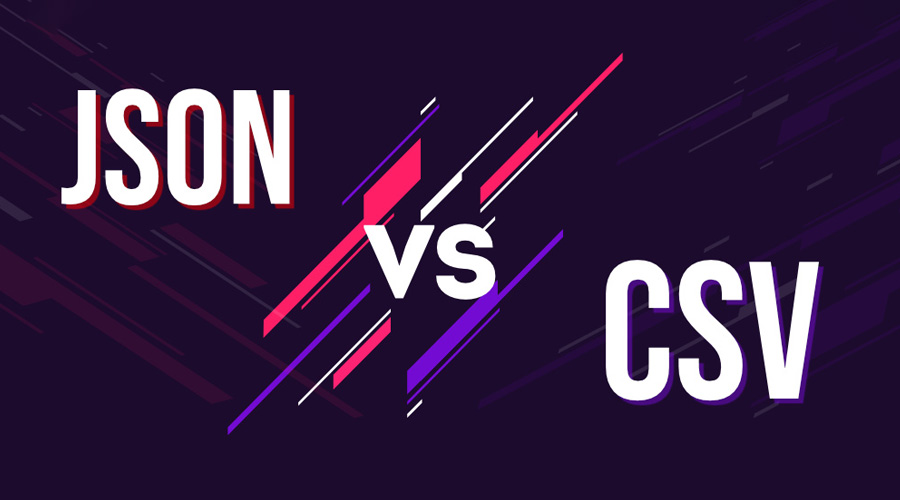
There are a number of packages that convert Python JSON to CSV. Two popular file formats for transferring and storing data are JSON and CSV. While CSV is a plain-text format that represents tabular data with comma-separated values and is frequently used for data storage in spreadsheets and databases, JSON is a lightweight, human-readable format that is used for sending data between servers and web applications.
Python has a number of packages that make the JSON to CSV conversion process easier. With just a few lines of code, you may finish the conversion operation by utilizing these libraries.
How to Convert JSON to CSV in Python
To convert Python JSON to CSV in Python, you can follow these general steps:
- Read the JSON Data: Read the JSON data using Python’s
json
module or by loading it from a JSON file. This step is necessary to parse the JSON data and work with it in Python. - Convert to a DataFrame (Optional): You can convert the JSON data to a Pandas DataFrame if you want to perform data manipulation and analysis. Converting to a DataFrame is optional but can be useful.
- Write to CSV: Use the Pandas library to write the data (either the DataFrame or the parsed JSON data) to a CSV file.
Here’s a step-by-step example of how to convert JSON to CSV:
import json
import pandas as pd# Step 1: Read the JSON data (from a JSON file or a JSON string)
# Example 1: Reading from a JSON file
with open(‘data.json’, ‘r’) as json_file:
json_data = json.load(json_file)# Example 2: Reading from a JSON string (replace this with your JSON data)
json_data = ”’
[
{“name”: “John”, “age”: 30, “city”: “New York”},
{“name”: “Alice”, “age”: 25, “city”: “Los Angeles”}
]
”’# Parse the JSON data
data = json.loads(json_data)# Step 2 (Optional): Convert to a Pandas DataFrame
df = pd.DataFrame(data)# Step 3: Write the DataFrame or JSON data to a CSV file
# If using a DataFrame:
df.to_csv(‘output.csv’, index=False)# If using the parsed JSON data (without a DataFrame):
with open(‘output.csv’, ‘w’) as csv_file:
csv_file.write(‘name,age,city\n’) # Write header row
for item in data:
csv_file.write(f'{item[“name”]},{item[“age”]},{item[“city”]}\n’)print(“JSON data has been converted to CSV.”)
After running this code, you will have your JSON data converted into a CSV file named output.csv
. Make sure to adjust the code to match the structure and source of your JSON data.
CSV or JSON, which Format is better for your AI training data?
With today’s technology, handling vast volumes of data is necessary. The performance of your system and project expenses can be maximized by choosing the appropriate data format. Building algorithms, creating apps, programming machine learning features, and training artificial intelligence all require data. To obtain useful insights, you require data storage formats that are simple to use, store, transfer, retrieve, and alter. Use the appropriate data format when exporting your excel sheet to a data file, such as MySQL. The most widely used data formats are JSON, XML, PARQUET, CSV, and AVRO.
The choice between Python JSON to CSV as the format for AI training data depends on various factors, including the nature of your data, the tools and libraries you’re using for AI, and your specific use case. Both formats have their advantages and disadvantages:
CSV (Comma-Separated Values):
- Structured Data: CSV is excellent for structured data where each row represents an example or data point, and columns represent features or attributes. This format is commonly used for tabular data.
- Simplicity: CSV files are straightforward and easy to work with. They are lightweight, and most programming languages provide built-in support for reading and writing CSV data.
- Efficiency: CSV can be more space-efficient compared to JSON, as it doesn’t include extensive formatting or metadata.
- Interoperability: CSV files can be easily imported into various data analysis and machine learning tools, such as pandas in Python.
JSON (JavaScript Object Notation):
- Hierarchical and Semi-Structured Data: JSON is versatile and suitable for semi-structured or hierarchical data, where you have nested structures, varying attributes, or non-tabular data.
- Metadata: JSON allows for including metadata and more complex data structures. It’s flexible and can represent more than just tabular data.
- Human-Readable: JSON is human-readable and is often used when data needs to be both machine-readable and easily understandable by humans.
- Compatibility with Web APIs: JSON is commonly used for exchanging data with web APIs, making it a natural choice for AI applications that involve web services.
- No Need for a Schema: JSON doesn’t require a predefined schema, making it more adaptable to changing data structures.
How to Convert JSON to CSV using Python Libraries
You can convert JSON data to CSV format in Python using various libraries, but one of the most commonly used libraries for this purpose is pandas
. Here’s a step-by-step guide on how to convert JSON to CSV using pandas
:
1. Install pandas: If you haven’t already installed pandas, you can do so using pip:
pip install pandas
2. Import the necessary libraries:
import pandas as pd
3. Read JSON data:
You need to read your JSON data into a Pandas DataFrame. You can use the pd.read_json()
function for this. You can pass the JSON data as a file or as a JSON string.
If your JSON data is in a file:
df = pd.read_json(‘your_data.json’)
If you have JSON data as a string:
import json
json_data = ”’
[
{“name”: “John”, “age”: 30, “city”: “New York”},
{“name”: “Alice”, “age”: 25, “city”: “Los Angeles”}
]
data = json.loads(json_data)
df = pd.DataFrame(data)
4. Write the DataFrame to a CSV file:
Now that you have your JSON data in a DataFrame, you can use the Python JSONto_csv()
function to write it to a CSV file.
df.to_csv(‘output.csv’, index=False)
The index=False
argument prevents writing the DataFrame index to the CSV file.
Here’s the complete code for converting JSON to CSV:
import pandas as pd
# Read JSON data (choose one of the methods)
# If data is in a file:
# df = pd.read_json(‘your_data.json’)# If data is a JSON string:
json_data = ”’
[
{“name”: “John”, “age”: 30, “city”: “New York”},
{“name”: “Alice”, “age”: 25, “city”: “Los Angeles”}
]
”’
data = json.loads(json_data)
df = pd.DataFrame(data)# Write DataFrame to a CSV file
df.to_csv(‘output.csv’, index=False)
After running this code, you will have your JSON data converted into a CSV file named output.csv
.
How to Read JSON Data Using Pandas
Reading the JSON data is the next step after importing the libraries. A pandas DataFrame can be created from a JSON string or file using the pd.read_json() function.
You can read JSON data into a Pandas DataFrame using the pandas.read_json()
function. Here’s how to do it:
1. Import the necessary libraries:
First, make sure you’ve imported the pandas
library.
import pandas as pd
2. Read JSON data:
You can read JSON data from a file or from a JSON string.
a. Read JSON data from a file:
If your JSON data is in a file, you can use pd.read_json()
like this:
df = pd.read_json(‘your_data.json’)
Replace 'your_data.json'
with the path to your JSON file.
b. Read JSON data from a JSON string:
If you have JSON data as a string, you can use pd.read_json()
with a JSON string as follows:
json_data = ”’
{
“name”: “John”,
“age”: 30,
“city”: “New York”
}
”’
df = pd.read_json(json_data, orient=’index’)
You may need to specify the orient
parameter based on the structure of your JSON data. The example above assumes that the JSON data is in a dictionary format.
Here’s the complete code for reading JSON data into a Pandas DataFrame:
After running this code, you will have your JSON data loaded into a Pandas DataFrame named df
, which you can then manipulate and analyze using Pandas functions.
How to Write to CSV With Pandas
You can write data to a CSV file using Pandas by using the to_csv()
method provided by Pandas DataFrames. Here’s how to convert Python JSON to CSV pandas, you can follow these steps:
1. Import the necessary libraries:
First, make sure you’ve imported the pandas
library.
import pandas as pd
2. Create or obtain a Pandas DataFrame:
You’ll need a Pandas DataFrame that contains the data you want to write to a CSV file. If you don’t have a DataFrame, you can create one by reading data from various sources, such as JSON, and SQL databases, or by manually constructing it from Python lists or dictionaries.
Here’s an example of creating a simple DataFrame:
data = {
‘Name’: [‘John’, ‘Alice’, ‘Bob’],
‘Age’: [30, 25, 35],
‘City’: [‘New York’, ‘Los Angeles’, ‘Chicago’]
}df = pd.DataFrame(data)
Write the DataFrame to a CSV file:
Once you have your DataFrame ready, you can use the to_csv()
method to write it to a CSV file.
df.to_csv(‘output.csv’, index=False)
- The
to_csv()
function takes the filename as its first argument and settingindex=False
prevents writing the DataFrame index as a column in the CSV file.
Here’s the complete code for writing a Pandas DataFrame to a CSV file:
import pandas as pd
# Create or obtain a Pandas DataFrame
data = {
‘Name’: [‘John’, ‘Alice’, ‘Bob’],
‘Age’: [30, 25, 35],
‘City’: [‘New York’, ‘Los Angeles’, ‘Chicago’]
}
df = pd.DataFrame(data)# Write the DataFrame to a CSV file
df.to_csv(‘output.csv’, index=False)
After running this code, you will have your DataFrame data written to a CSV file named output.csv
in the current working directory. You can specify a different path for the output file if needed.
Python JSON to CSV column order:
When you convert JSON to CSV using Pandas in Python, the order of columns in the resulting CSV file is typically determined by the order of the columns in the DataFrame. However, if you want to specify a specific order for the columns in the CSV file, you can do so by reordering the columns in the DataFrame before writing it to the CSV file. Here’s how you can do that:
- Create a Pandas DataFrame from your JSON data.
- Reorder the columns as desired using DataFrame indexing.
- Use the
to_csv()
method to write the DataFrame to a CSV file with the desired column order.
Here’s an example of how to reorder columns in a Pandas DataFrame before converting it to a CSV file:
import pandas as pd
# Create a DataFrame from JSON data
data = {
‘Name’: [‘John’, ‘Alice’, ‘Bob’],
‘Age’: [30, 25, 35],
‘City’: [‘New York’, ‘Los Angeles’, ‘Chicago’]
}
df = pd.DataFrame(data)# Define the desired column order
desired_column_order = [‘Name’, ‘City’, ‘Age’]# Reorder the columns based on the desired order
df = df[desired_column_order]# Write the DataFrame to a CSV file
df.to_csv(‘output.csv’, index=False)
In this example, we first create a DataFrame, then reorder the columns based on the desired_column_order
list, and finally, write the DataFrame to a CSV file. The resulting CSV file will have columns in the specified order.
JSON to CSV Online
If you have JSON data that you want to convert to CSV format online, you can use various web-based tools and services. Here are a few online Python JSON to CSV converters you can use:
Convert Python JSON to CSV Online Tool:
JSON to CSV Converter (Convert JSON to Excel):
- JSON to CSV Converter
JSON to CSV Online Converter:
- JSON to CSV Online Converter
JSON to CSV Python :
pandas
library. First, import pandas
. Then, read your JSON data using pd.read_json()
a file or a JSON string. Optionally, manipulate the data with Pandas. Finally, write it to a CSV file using to_csv()
. Ensure to specify the filename and use index=False
to prevent including the DataFrame index. This process simplifies the conversion of structured JSON data into a tabular CSV format for analysis or sharing.To convert JSON to CSV in Python using the pandas
library, follow these steps. First, import pandas
. Next, read your JSON data with pd.read_json()
, which can parse a JSON file or JSON data in string format into a DataFrame. You can manipulate the data if necessary using Pandas functions. Afterward, use the to_csv()
method to write the DataFrame to a CSV file. Specify the filename as the argument and set index=False
it to exclude writing the DataFrame index. This process streamlines the conversion of JSON data into a tabular CSV format, making it suitable for data analysis, reporting, or sharing with others.
Data can be sent and stored using the JSON syntax. Text created in JavaScript object notation is called JSON. Working with JSON data in Python is possible thanks to the JSON package, which is part of the language.