Assignment Operator in Python: Variables and Values
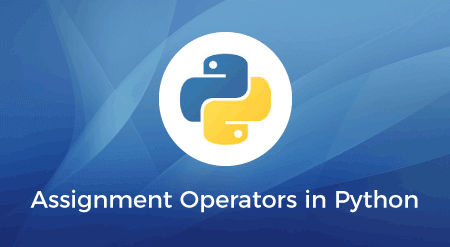
The assignment operator in Python (=
) serves as the cornerstone of variable management and data storage. It enables you to associate variables with values, creating a foundation for all your programming endeavors. Whether you’re assigning integers, strings, or the results of complex expressions, this operator plays a pivotal role in the clarity and simplicity of Python code. Understanding its syntax and versatility empowers you to manipulate data efficiently, making it a vital concept for beginners and experienced programmers alike.
Assignment Operator in Python: Variables and Values
In Python, as in many programming languages, the assignment operator is a fundamental concept that allows you to store and manipulate data. It is denoted by the equals sign=
and plays a central role in variable creation and value assignment. This article will delve into the assignment operator, how it works, and its importance in Python programming.
- The simple assignment operator ( = )
- Add an equal operator ( += )
- Subtract and equal operators ( -= )
- Asterisk and equal operator ( *= )
- Divide and equal operator ( /= )
- Modulus and equal operator ( %= )
- Double divide and equal operator ( //= )
Variables and Values
In Python, variables are used to store data. Think of a variable as a container that holds a specific value. The value can be of various types, such as numbers, strings, lists, or even more complex data structures.
Here’s how you can create a variable and assign a value to it using the assignment operator:
x = 42 # Assign the integer value 42 to the variable ‘x’
name = “John” # Assign the string value “John” to the variable ‘name’
In these examples, we’ve used the assignment operator (=
) to associate the variable name (x
and name
) with a particular value.
How the Assignment Operator Works
The assignment operator works by taking the value on the right side and associating it with the variable on the left side. It does not mean “equals” in a mathematical sense but should be read as “gets assigned to.” This means the value on the right is stored in the variable on the left.
Let’s illustrate this with an example of the assignment operator in Python:
x = 10
y = x
In this example, x
is assigned the value 10
, and then y
is assigned the value of x
. After these assignments, both x
and y
hold the value 10
.
Mutability and Immutability
The behavior of the assignment operator can vary depending on the type of data being assigned. Some data types in Python are mutable, which means they can be changed in place, while others are immutable, which means they cannot be changed.
For example, when dealing with lists, which are mutable in assignment operator in Python:
list1 = [1, 2, 3]
list2 = list1 # Both variables refer to the same list
list2.append(4) # Modifies the original list
print(list1) # Output: [1, 2, 3, 4]
In contrast, immutable types like strings cannot be modified in place:
str1 = “hello”
str2 = str1 # Both variables refer to the same string
str2 += ” world” # Creates a new string and assigns it to ‘str2’
print(str1) # Output: “hello”
Understanding mutability and immutability is crucial when working with Python variables.
Reassignment
Variables can be reassigned multiple times, changing the value they hold.
x = 5
x = x + 1 # Reassigning ‘x’ with a new value
In this example, x
is initially assigned the value5
, and then it’s reassigned to the result ofx + 1
, which is6
.
Conclusion
The assignment operator in Python is a fundamental concept that enables you to create variables and associate them with values. It’s essential to grasp how it works, understand mutability and immutability, and be familiar with variable reassignment. With this knowledge, you can effectively manipulate data in Python and build powerful programs.
Remember that Python’s simplicity and readability are key reasons for its popularity, and the assignment operator is a core element of that simplicity. It allows you to express your intentions clearly and concisely in your code.
Types of assignment operator in Python:
In Python, the primary assignment operator is the equals sign =
. It is used for assigning values to variables. Here are some common assignment operators in Python:
1. Simple Assignment (=
): Used to assign a value to a variable.
x = 10 # Assign the value 10 to the variable ‘x’
2. Addition Assignment (+=
): Adds the right-hand value to the left-hand variable and assigns the result to the variable.
x = 5
x += 2 # x is now 7
-=
): Subtracts the right-hand value from the left-hand variable and assigns the result to the variable.x = 10
x -= 3 # x is now 7
4. Multiplication Assignment (*=
): Multiplies the left-hand variable by the right-hand value and assigns the result to the variable.
x = 3
x *= 4 # x is now 12
5. Division Assignment (/=
): Divides the left-hand variable by the right-hand value and assigns the result to the variable.
x = 20
x /= 5 # x is now 4.0
6. Modulus Assignment (%=
): Calculates the modulus of the left-hand variable with the right-hand value and assigns the result to the variable.
x = 13
x %= 5 # x is now 3
7. Exponentiation Assignment (**=
): Raises the left-hand variable to the power of the right-hand value and assigns the result to the variable.
x = 2
x **= 3 # x is now 8
8. Floor Division Assignment (//=
): Performs floor division on the left-hand variable by the right-hand value and assigns the result to the variable.
x = 10
x //= 3 # x is now 3
These assignment operators make it convenient to perform arithmetic operations and update variables in a concise manner. They are commonly used in Python for a wide range of programming tasks.
What is a floor division assignment operator in Python?
The purpose of the floor division assignment operator (//=
) in Python is to perform floor division on a variable and then update the variable’s value with the result of the floor division. Floor division is a division where the result is rounded down to the nearest integer that is less than or equal to the true mathematical result. This operator is useful for various reasons:
- Handling Division with Remainders: Floor division is often used when you want to divide a value into equal parts, and you need to know how many whole parts you can obtain. It’s particularly useful when dealing with situations where fractional parts are not meaningful or cannot be used.
- Data Manipulation: Floor division can be essential in data manipulation and analysis, such as grouping data into bins or partitions. It ensures that each value is associated with a specific category or range.
- Mathematical Operations: In mathematical and scientific calculations, floor division is used to calculate quantities like the number of integer solutions to an equation or to perform integer division in modular arithmetic.
Here’s a practical example:
total_distance = 100
distance_per_hour = 30
hours_travelled = total_distance // distance_per_hour
In this example, the floor division operator is used to calculate the number of hours required to cover a total distance of 100 units when traveling at a rate of 30 units per hour. The result hours_travelled
is assigned a value, representing that it takes 3 full hours to travel the distance.
The floor division assignment operator (//=
) is especially useful when you want to perform floor division and immediately update a variable’s value, saving you the extra step of reassigning the result to the same variable.
Shorthand assignment operators in Python are used to combine an operation with an assignment in a concise and readable way. They perform an operation on a variable and update the variable with the result of that operation. Here are the common shorthand assignment operators in Python:
- Addition Assignment (
+=
): Adds the right-hand value to the left-hand variable and assigns the result to the variable. - Subtraction Assignment (
-=
): Subtracts the right-hand value from the left-hand variable and assigns the result to the variable. - Multiplication Assignment (
*=
): Multiplies the left-hand variable by the right-hand value and assigns the result to the variable. - Division Assignment (
/=
): Divides the left-hand variable by the right-hand value and assigns the result to the variable. - Multiplication Assignment (
*=
): Multiplies the left-hand variable by the right-hand value and assigns the result to the variable. - Modulus Assignment (
%=
): Calculates the modulus of the left-hand variable with the right-hand value and assigns the result to the variable. - Exponentiation Assignment (
**=
): Raises the left-hand variable to the power of the right-hand value and assigns the result to the variable. - Floor Division Assignment (
//=
): Performs floor division on the left-hand variable by the right-hand value and assigns the result to the variable.
Shorthand assignment operators are a convenient way to both perform an operation and update a variable’s value in one step. They are often used in loops, mathematical calculations, and data manipulation to make code more concise and efficient.
Understanding Assignment Operator in Python String:
In Python, the assignment operator (=
) is used to assign values to variables, including string variables. Here’s how it works for assigning values to string variables:
text = “Hello, World!” # Assign the string “Hello, World!” to the variable ‘text’
In this example, the assignment operator is used to create a string variable named text
and assign it the value “Hello, World!”. The variabletext
now holds this string, allowing you to access and manipulate it in your code.
You can reassign a string variable with a new value:
text = “Python is great!” # Reassign ‘text’ with a new string value
The assignment operator is not limited to just creating string variables; it is used to assign values to variables of various data types, including numbers, lists, dictionaries, and more. It plays a fundamental role in Python for storing and managing data.
Understanding Assignment Operator in Python Syntax:
The assignment operator in Python, denoted by the equals sign (=
), is used to assign values to variables. The syntax for using the assignment operator is straightforward:
variable_name = value
Here’s a breakdown of the assignment operator in Python syntax:
- Variable Name: This is an identifier that you choose to represent a variable. It can be almost any combination of letters, numbers, and underscores, but it must start with a letter or underscore. Variable names are case-sensitive, so “myVar” and “myvar” are considered different variables.
- Equals Sign (=): The equals sign is the assignment operator. It is used to assign a value to the variable on the left.
- Value: This is the actual data that you want to store in the variable. It can be a literal value (e.g., numbers, strings, lists) or the result of an expression.
Here are some examples to illustrate the assignment operator in Python syntax:
# Assigning an integer to a variable
x = 42# Assigning a string to a variable
name = “Alice”# Assigning the result of an expression to a variable
result = 2 * (x + 5)
Once a value is assigned to a variable, you can use the variable in your code to access and manipulate that value. The assignment operator in Python plays a central role in Python programming, allowing you to work with data efficiently.
See Also:
- Unveiling the Distinctions: Web Crawler vs Web Scrapers
- How to Make Money with Web Scraping Using Python
- How to Type Cast in Python with the Best 5 Examples
- Best Variable Arguments in Python
- 5 Best AI Prompt Engineering Certifications Free
- 5 Beginner Tips for Solving Python Coding Challenges
- Exploring Python Web Development Example Code
- “Python Coding Challenges: Exercises for Success”
- ChatGPT Prompt Engineering for Developers:
- How to Make AI-Generated Video|AI video generator
- 12 Best Python Web Scraping Tools and Libraries with Pros & Cons
- 7 Best Python Web Scraping Library To Master Data Extraction
- Explore the World of Character AI Generator
- Boolean Operators in Python Examples (And|Or|Not)
- Python List Slicing: 3 Best Advanced Techniques