5 Beginner Tips for Solving Python Coding Challenges:
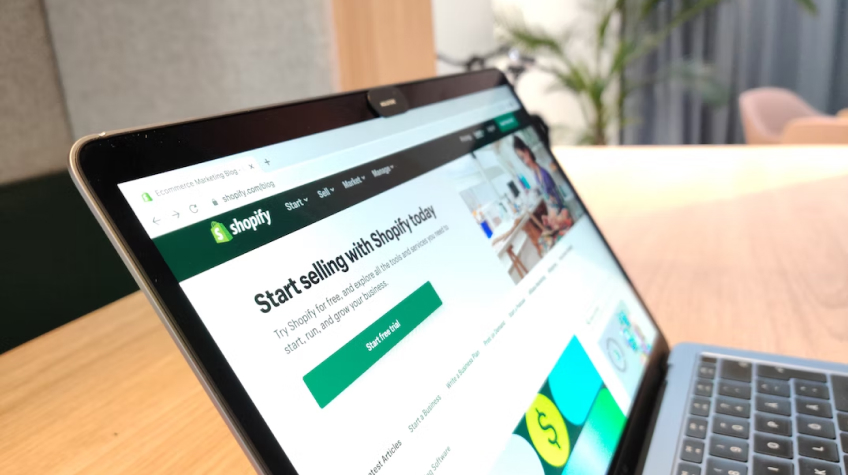
“Welcome to our Python Coding Challenges blog, where we embark on a journey of problem-solving excellence! Whether you’re preparing for technical interviews or simply enhancing your programming skills, our blog is your go-to resource. Discover valuable tips, expert strategies, and unique exercises designed to unravel the intricacies of coding challenges. Join us as we delve into the world of algorithms, data structures, and efficient coding practices. Let’s elevate your Python programming prowess and conquer every coding challenge that comes your way!”
Coding challenges come in various types, covering a wide range of topics and difficulty levels. Here are some common types of Python coding challenges:
- Algorithmic Challenges:
- Sorting Algorithms: Implementing sorting algorithms like Bubble Sort, Merge Sort, or Quick Sort
- Searching Algorithms: Solving problems that involve searching algorithms, such as binary search
- Data Structure Challenges:
- Linked Lists: Implementing and manipulating singly or doubly linked lists
- Trees: Solving problems related to binary trees, binary search trees, or other tree structures
- Graphs: Implementing graph algorithms or solving problems related to graphs
- String Manipulation Challenges:
- String Reversal: Reversing a string using different approaches
- Anagram Detection: Checking if two strings are anagrams of each other.
- Substring Search: Implementing algorithms for substring search
- Dynamic Programming Challenges:
- Fibonacci Sequence: Solving problems related to the Fibonacci sequence using dynamic programming
- Longest Common Subsequence: Finding the longest common subsequence in two strings
- Array Challenges:
- Array Rotation: Rotating elements in an array
- Maximum Subarray Sum: Finding the maximum sum of a subarray in an array
- Mathematical Challenges:
- Prime Number Generation: Implementing algorithms to generate prime numbers
- Factorial Calculation: Efficiently calculating the factorial of a number
- Recursion Challenges:
- Recursive Functions: Solving problems using recursive algorithms
- Tower of Hanoi: Implementing the Tower of Hanoi problem using recursion
- Graph Algorithms:
- Breadth-First Search (BFS): Implementing BFS on a graph
- Depth-First Search (DFS): Solving problems using DFS on a graph
- Greedy Algorithms:
- Fractional Knapsack Problem: Solving optimization problems using a greedy approach
- Activity Selection Problem: Selecting a maximum set of activities without conflicts.
- Bit Manipulation Challenges:
- Bitwise Operations: Solving problems that involve bitwise manipulation
- Counting Set Bits: Counting the number of set bits in a binary representation
- Dynamic Programming Challenges:
- 0/1 Knapsack Problem: Solving the classic 0/1 knapsack optimization problem
- Coin Change Problem: Finding the number of ways to make change using a given set of coins
- Backtracking Challenges:
- N-Queens Problem: Placing N queens on a chessboard without any two queens attacking each other.
- Sudoku Solver: Implementing a program to solve Sudoku puzzles.
- Project Euler Problems:
- Solving mathematical and computational problems from the Project Euler archive, which often involves a combination of mathematical insight and programming skills.
- Coding Challenges from Online Platforms:
- Solving challenges from platforms such as LeetCode, HackerRank, CodeSignal, or Codewars, which cover a wide range of topics and difficulty levels.
These are just a few examples, and the world of Python coding challenges is vast and diverse. The key is to explore challenges that align with your current skill level and gradually take on more complex problems as you grow as a programmer, solving Python coding challenges:
Solving coding challenges can be both rewarding and challenging. Here are some tips to help you tackle coding challenges more effectively:
- Understand the Problem:
- Carefully read and understand the problem statement.
- Identify the input requirements, constraints, and expected output.
- Plan Before Coding:
- Break down the problem into smaller, manageable tasks.
- Consider different approaches and algorithms before starting to code.
- Write pseudocode or outline your solution to guide your implementation.
- Handle Edge Cases:
- Consider edge cases and corner scenarios in your solution.
- Ensure your solution works for the minimum and maximum possible input values.
- Write Modular Code:
- Divide your solution into modular functions or methods.
- Each function should have a clear purpose and be focused on a specific task.
- Follow Pythonic Conventions:
- Adhere to Python coding conventions for readability.
- Use meaningful variable and function names.
- Employ proper indentation and whitespace.
- Optimize for Time and Space Complexity:
- Strive for efficient algorithms to optimize time complexity.
- Be mindful of space complexity, especially in scenarios with large datasets.
- Consider trade-offs between time and space.
- Test Thoroughly:
- Implement unit tests to verify the correctness of your solution.
- Validate your code with diverse inputs, ensuring to include edge cases in your testing.
- Consider creating a test suite to automate testing.
- Debugging:
- If your solution is not working as expected, use print statements or a debugger to identify issues.
- Step through your code to understand its flow and identify logical errors.
- Learn from Mistakes:
- If your solution is incorrect, analyze why it failed.
- Understand the underlying concept or bug that caused the error.
- Use mistakes as learning opportunities for improvement.
- Efficient Data Structures:
- Choose the appropriate data structures for the problem.
- Leverage built-in Python data structures such as lists, sets, dictionaries, and queues.
- Explore Python Libraries:
- Utilize relevant Python libraries and modules to simplify your code.
- Familiarize yourself with libraries such as itertools, collections, and math.
- Ask for Help and Feedback:
- Seek help from online forums or communities when you’re stuck.
- Share your solutions and seek constructive feedback from others.
- Time Management in Interviews:
- Practice solving problems under time constraints.
- Develop strategies to manage your time effectively during technical interviews.
- Stay Consistent:
- Regularly practice coding challenges to stay sharp.
- Consistency is key to improvement, so make coding challenges a part of your routine.
- Read and Learn:
- After solving a challenge, read editorial solutions or discussions to learn alternative approaches.
- Understand different perspectives and solutions to broaden your problem-solving skills.
Remember, coding challenges are not just about finding a solution; they’re an opportunity to enhance your problem-solving skills and become a more proficient Python programmer. Practice regularly, and with time, you’ll become more comfortable tackling a wide range of challenges.
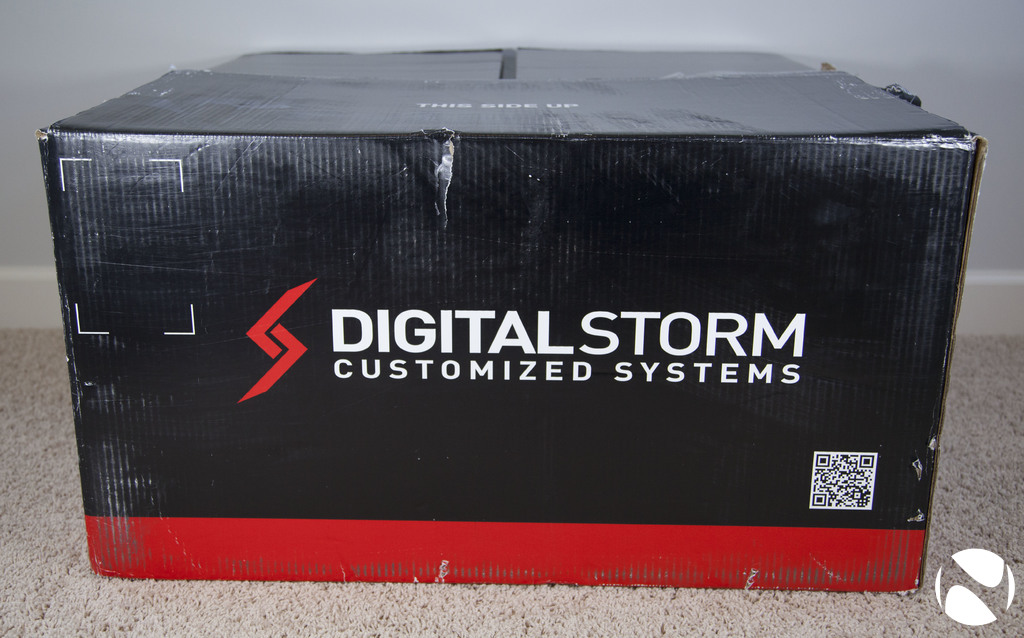
Benefits of Python Coding Challenges:
A. Skill improvement
1. Enhancing problem-solving abilities
. Strengthening algorithmic thinking
B. Interview preparation
1. Commonly tested concepts
2. How coding challenges reflect real-world problem-solving
C. Learning Python features
1. Exploring various Python libraries and modules
2. Utilizing built-in functions for efficient solutions
Types of Python Coding Challenges
A. Online platforms for practicing challenges
1. CodeSignal
2. LeetCode
3. HackerRank
4. Codewars
B. Example challenges and their difficulty levels
1. Easy challenges
2. Medium challenges
3. Hard challenges
Preparing for advanced Python coding challenges:
Preparing for advanced Python coding challenges in technical interviews requires a strategic approach to ensure success. Follow these steps to effectively crack your next Python coding interview:
- Solidify Data Structure Knowledge:
- Strengthen your understanding of fundamental data structures such as lists, dictionaries, and tuples. Practice custom implementations of complex structures like LinkedLists to showcase a deep understanding.
- Whiteboard Practice:
- Prepare for whiteboarding interview rounds by practicing writing Python constructs, including loops and control flow models, on a whiteboard or paper. Many top companies use whiteboarding as part of their interview process.
- Front-End Basics:
- Gain a basic understanding of front-end technologies like HTML, JavaScript, and CSS. While Python may be the primary focus, a holistic knowledge of web technologies can be beneficial in certain technical interviews.
- OOPs Concepts:
- Ensure a clear grasp of Object-Oriented Programming (OOPs) concepts. Understand how to implement and use classes, inheritance, encapsulation, and polymorphism in Python.
- Mock Interviews with Experts:
- Engage in mock interviews with industry experts to simulate real interview conditions. This practice helps alleviate interview anxiety, builds confidence, and identifies areas for improvement.
- Strategic Problem Solving:
- Approach each coding question strategically. Gain a comprehensive understanding of the problem before delving into the code implementation. Initially, use a brute force approach and then optimize your solution, considering factors like time complexity.
- Code Implementation:
- Code your solution only after a comprehensive understanding of the problem and careful consideration of optimization. Strive for the most efficient solution possible, and be ready to justify your choices during the interview.
- Think Aloud:
- Practice thinking aloud while solving problems. Articulate your thought process and approach to the recruiters. This not only demonstrates your problem-solving skills but also provides insights into your decision-making.
- Practice with Google’s Coding Challenges:
- Familiarize yourself with Google’s coding challenges. These challenges are known for their complexity and can be excellent preparation for tackling advanced problems in technical interviews.
Remember, consistent practice and thoughtful preparation are key to success in advanced Python coding challenges. Each step in your preparation journey contributes to your overall readiness for technical interviews. Stay focused, practice regularly, and approach challenges with a strategic mindset.
Conclusion:
Python coding challenges hold immense significance in shaping and enhancing programming skills. These challenges serve as a dynamic training ground, fostering problem-solving abilities, algorithmic thinking, and proficiency in Python. They are not merely exercises but rather strategic tools that prepare individuals for real-world projects and technical interviews. Through coding challenges, one can develop the versatility needed to navigate diverse domains within the tech industry and stay competitive in a rapidly evolving landscape.
As you embark on your coding journey, remember that improvement is a continuous process. Embrace the challenges, both small and complex, as opportunities to refine your skills and broaden your understanding of Python.
I encourage you to share your feedback, insights, and personal experiences with the community. Whether you’ve faced challenges, discovered efficient solutions, or learned valuable lessons, your input contributes to a collaborative learning environment.
See Also:
-
- Unveiling the Distinctions: Web Crawler vs Web Scrapers
- How to Make Money with Web Scraping Using Python
- How to Type Cast in Python with the Best 5 Examples
- Best Variable Arguments in Python
- 5 Best AI Prompt Engineering Certifications Free
- 5 Beginner Tips for Solving Python Coding Challenges
- Exploring Python Web Development Example Code
- “Python Coding Challenges: Exercises for Success”