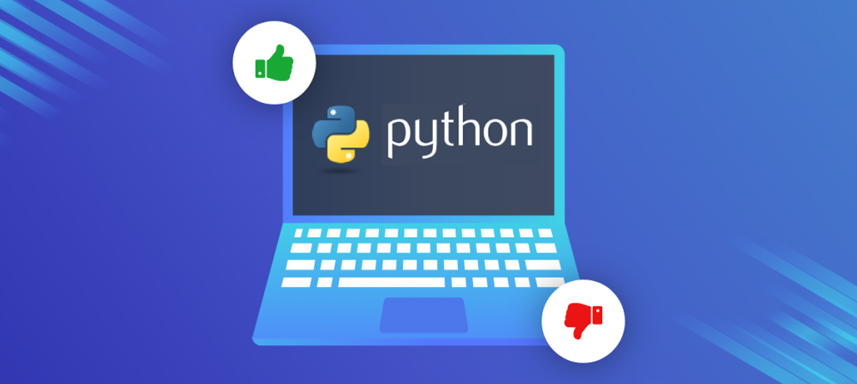
Python scopes are a fundamental concept governing the accessibility and visibility of variables and functions within your code. In Python, scope refers to the visibility and accessibility of variables, objects, and functions within a program. Understanding scope is crucial for writing clean and maintainable code. This blog post will provide a comprehensive overview of scope in Python, covering global scope, local scope, nested scope, and the concept of variable shadowing. Let’s dive in!
What is Scope in Python: Definition and Characteristics?
Python scope is a fundamental concept in Python that governs the accessibility and visibility of variables and functions within your code. This comprehensive guide will provide an in-depth understanding of scope in Python, covering global scope, local scope, nested scope, variable shadowing, and best practices for managing scope. Let’s dive in and explore this essential aspect of Python programming.
1. Global Scope:
In Python, global scope refers to the outermost scope in your code. Variables defined in the global scope are accessible from anywhere within the module or script. Here are the key points regarding global scope:
- Definition and Characteristics:
- Global scope is the highest level of visibility in Python.
- Variables defined outside of any function or method have a global scope.
- Global variables can be accessed and modified from any part of the program.
- Accessing Global Variables:
- Global variables can be directly accessed within any function or block of code.
- They are accessible by their variable name without any special syntax or keyword.
- Modifying Global Variables:
- Global variables can be modified from any part of the program that has access to them.
- Changes made to a global variable within a function will affect its value throughout the program.
- Global Keyword:
- The global keyword allows you to explicitly declare a variable as global within a function.
- This is required if you want to modify a global variable from within a function’s local scope.
- By using the global keyword, you inform Python that the variable being referenced or modified is the global one, not a new local variable with the same name.
The syntax for declaring a global variable using the global keyword is:
global variable_name
Example:
count = 0 # Global variable
def increment():
global count # Declare count as a global variable
count += 1
print(count)
increment() # Output: 1
increment() # Output: 2
By using the global keyword, you ensure that the count variable refers to the global variable and increments its value rather than creating a new local variable within the function.
Understanding global scope and effectively using the global keyword enable you to access and modify variables from anywhere in your program. However, it is generally recommended to minimize the use of global variables and prefer passing values as function arguments to maintain code readability and reduce potential issues related to variable Python scope.
2. Local Scope:
Local scope in Python refers to the innermost scope, specific to a function or method. It is created when a function is called and exists only within that function or method. Here are the key points regarding local scope:
- Introduction to Local Scope and Functions:
- Local scope is associated with functions and methods.
- When a function or method is called, a local scope is created for that particular invocation.
- The purpose of local scope is to provide a separate namespace for variables within the function.
- Variables Defined within a Function:
- Variables defined inside a function are called local variables.
- Local variables are accessible and visible only within the function where they are defined.
- They cannot be directly accessed from outside the function.
- Limited Accessibility of Local Variables:
- Local variables are confined to the Python scope of the function or method in which they are defined.
- They cannot be accessed or modified from outside the function.
- Attempting to access a local variable from outside the function will result in a NameError.
- Lifetime of Local Variables:
- Local variables have a limited lifetime.
- They are created when the function or method is called and exist only during the execution of that function.
- Once the function or method finishes executing, local variables are destroyed, and their memory is released.
Example:
def example_function():
local_variable = "I am a local variable" # Local variable
print(local_variable)
example_function() # Output: "I am a local variable"
print(local_variable) # Raises NameError: Name 'local_variable' is not defined
In the above example, local_variable
is defined within the example_function()
. It is accessible and can be used within the function, but trying to access it outside the function will result in a NameError.
Understanding local scope is crucial for writing modular and encapsulated code. It allows you to create variables that are only relevant and accessible within the context of a specific function or method. Remember that local variables have a limited lifetime and are destroyed once the function finishes executing.
In Python, nested Python scope refers to the ability to define functions within other functions. This creates a hierarchy of scopes, where each nested function has access to variables from its own scope as well as the scopes of its enclosing functions. Here are the key points regarding nested scope:
- Functions defined within functions:
- Python allows defining functions inside other functions, resulting in nested scope.
- The inner function is called a nested function or an inner function, while the outer function is referred to as the enclosing function.
- Accessing Variables from Outer Scopes:
- In nested scope, an inner function has access to variables from its own scope as well as the Python scopes of its enclosing functions.
- This means the inner function can access and use variables defined in its own scope, the enclosing function’s scope, and even the global scope.
- Concept of Closure:
- Closure is a powerful concept closely related to nested scope.
- A closure is formed when an inner function references a variable from its enclosing scope, even after the outer function has finished executing.
- This allows the inner function to “remember” and access the values of variables from the enclosing scope where it was defined.
- Practical Use of Closures:
- Closures are commonly used for creating functions with persistent states or for implementing data hiding and encapsulation.
- They are particularly useful when you want to create functions that retain access to certain variables or configurations, even after the original function has completed its execution.
Example:
def outer_function(x):
def inner_function(y):
return x + y # inner_function has access to x from outer_function's scope
return inner_function
add_5 = outer_function(5)
result = add_5(3) # Output: 8
The above example outer_function
defines an inner function called inner_function
. The inner function has access to the x
variable from the enclosing Python scope of outer_function
. When outer_function
is called with the argument 5, it returns inner_function
. The returned inner_function
retains access to the value of x
(which is 5) even after outer_function
has finished executing. Subsequently, calling add_5(3)
adds 3 to the remembered value x
and returns the result.
Understanding nested scopes and closures allows you to create more flexible and powerful functions. By leveraging the concept of closure, you can create functions that carry their own state or retain access to specific variables, enhancing the functionality and reusability of your code.
4. Variable Shadowing:
Variable shadowing occurs when a variable in an inner scope has the same name as a variable in an outer scope. This results in the inner variable “shadowing” or hiding the outer variable, making the outer variable inaccessible within the inner scope. Here are the key points regarding variable shadowing:
- Definition and Examples:
- Variable shadowing refers to the situation where a variable in an inner scope has the same name as a variable in an outer scope, causing the outer variable to be overshadowed or hidden.
- This can happen in nested functions, where a variable in an inner function has the same name as a variable in an outer function or the global scope.
- When a variable is shadowed, any reference to that variable within the inner Python scope will refer to the inner variable, not the outer one.
- Impact on Variable Accessibility:
- Variable shadowing affects the visibility and accessibility of variables.
- When a variable is shadowed, the inner variable takes precedence over the outer variable within the scope where the shadowing occurs.
- The outer variable becomes inaccessible within the inner scope, as it is overshadowed by the inner variable.
- Strategies for Avoiding Variable Shadowing:
- Use descriptive variable names: Choose meaningful and distinct names for your variables to minimize the chances of inadvertently shadowing variables.
- Avoid reusing variable names within nested scopes: Be mindful of the naming of variables in nested functions to prevent unintentional shadowing.
- Refactor code to reduce nesting: Excessive nesting can increase the likelihood of shadowing. Consider refactoring your code to reduce the levels of nesting, making it easier to manage variable names.
- Use function arguments and return values: Instead of relying on shadowing to access outer variables, pass values as arguments to inner functions and return values from inner functions to preserve clarity and maintain proper scoping.
Example:
x = 5 # Global variable
def outer_function():
x = 10 # Outer function's variable
def inner_function():
x = 15 # Inner function's variable
print(x) # Output: 15
inner_function()
print(x) # Output: 5
outer_function()
In the above example, the variable x
is shadowed within each nested scope. When inner_function
is called, it prints the value of the innermost variable,x
which is 15. However, outside the functions, the global variable x
remains unaffected and retains its value of 5.
To avoid variable shadowing and maintain code clarity:
- Use descriptive variable names to minimize naming conflicts.
- Be cautious when reusing variable names in nested Python scopes.
- Refactor the code to reduce excessive nesting.
- Prefer passing values as function arguments and returning values explicitly.
By following these strategies, you can minimize the chances of variable shadowing and improve the readability and maintainability of your code.
5. The global and nonlocal keywords are:
The global and nonlocal keywords are used in Python to access and modify variables in different Python scopes. Here are the key points regarding the global and nonlocal keywords:
- Global Keyword:
- The global keyword is used to explicitly declare a variable as global within a function.
- When a variable is declared as global, it can be accessed and modified within the function, affecting its global value outside the function.
- The global keyword is necessary when you want to modify a global variable from within a function’s local scope.
Example:
count = 0 # Global variable def increment(): global count # Declare count as a global variable count += 1 print(count) increment() # Output: 1 increment() # Output: 2 ``` In the above example, the global keyword is used to declare `count` as a global variable within the `increment()` function. This allows the function to access and modify the global `count` variable. Best Practice: - Minimize the use of global variables and prefer passing values as function arguments and returning values explicitly to maintain code clarity and reduce potential issues related to variable scope.
- Nonlocal Keyword:
- The nonlocal keyword is used to access and modify variables from enclosing (outer) scopes in nested functions.
- When a variable is declared as nonlocal, it allows the nested function to reference and modify that variable from the enclosing scope.
- The nonlocal keyword is necessary when dealing with nested functions that need to access and update variables from their enclosing Python scopes.
Example:
def outer_function(): x = 10 # Outer function's variable def inner_function(): nonlocal x # Declare x as a nonlocal variable x += 5 print(x) # Output: 15 inner_function() outer_function() ``` In the above example, the nonlocal keyword is used to declare `x` as a nonlocal variable within the `inner_function()`. This allows the inner function to access and modify the variable `x` from its enclosing scope. Best Practice: - Carefully consider the usage of nonlocal variables to ensure clear and maintainable code. Excessive reliance on nonlocal variables can lead to code that is harder to understand and debug.
Understanding the global and nonlocal keywords enables you to work with variables in different scopes effectively. However, it’s generally recommended to minimize the use of global and nonlocal variables for better code organization and maintainability.
6. Best Practices for Scope Management:
Managing Python scope effectively is crucial for writing clear, readable, and maintainable code. Here are some guidelines and best practices to follow when it comes to scope management:
- Minimize the Use of Global Variables:
- Global variables are accessible from anywhere in the code, which can make it difficult to track their modifications and understand their impact.
- Limit the use of global variables to only those cases where they are truly necessary.
- Instead, prefer passing values as function arguments and returning values explicitly to encapsulate data within functions and promote modular code.
- Favor Local Variables:
- Use local variables within functions whenever possible.
- Local variables have limited scope and are confined to the specific function or block where they are defined.
- They help maintain encapsulation, reduce naming conflicts, and make code more readable and self-contained.
- Choose Descriptive Variable Names:
- Use meaningful and descriptive names for your variables.
- Well-chosen variable names improve code readability and make it easier to understand the purpose and usage of variables.
- Avoid single-letter variable names or generic names that can lead to confusion or ambiguity.
- Avoid Naming Conflicts:
- Be mindful of naming conflicts, especially in nested Python scopes.
- Shadowing variables or using the same name for different variables can make code harder to understand and introduce unexpected behavior.
- Choose distinct and unambiguous names to minimize the chances of naming conflicts.
- Use Proper Scoping Techniques:
- Leverage function and block scoping to limit the visibility and accessibility of variables.
- Define variables in the narrowest scope possible, keeping them close to where they are used and minimizing their lifetime.
- Use nested functions and closures judiciously, ensuring that the scope hierarchy is well-managed and variables are accessed appropriately.
By following these best practices, you can improve the readability, maintainability, and reliability of your code. Proper Python scope management helps in avoiding naming conflicts, enhances code organization, and promotes modular and encapsulated design.
Conclusion:
Summarizing the key concepts covered in this article, highlighting the importance of understanding the scope of Python programming. Emphasizing the significance of proper scope management for writing clean, efficient, and bug-free code. Encouraging readers to apply the knowledge gained to improve their programming practices and create more robust Python applications.
What is Python variable scope?
In Python, variable scope determines the visibility and accessibility of variables within different parts of your code. It defines where a variable can be referenced and used. Python has three main types of variable Python scope:
- Local Scope:
- Variables defined within a function or method have local scope.
- Local variables are accessible only within the function or method where they are defined.
- Local variables are created when the function or method is called and cease to exist once the function or method finishes execution.
- Global Scope:
- Variables defined outside of any function or method have a global scope.
- Global variables can be accessed from anywhere within the module or script.
- Global variables maintain their value throughout the execution of the program.
- Enclosing (non-local) scope:
- In nested functions, the enclosing function’s scope acts as an enclosing scope.
- Variables defined in the enclosing function can be accessed by the nested functions.
- The enclosing scope bridges the gap between local and global scopes, allowing access to variables from the outer function.
Scope Resolution:
Python follows the LEGB rule for Python scope resolution:
- Local (L) scope: variables defined within the current function or method.
- Enclosing (E) scope: variables defined in the enclosing functions
- Global (G) scope: variables defined at the top level of the module.
- Built-in (B) scope: variables provided by Python’s built-in modules.
If a variable is not found in the local scope, Python searches for it in the enclosing, global, and built-in scopes in that order.
Variable Shadowing:
Variable shadowing occurs when a variable in an inner scope has the same name as a variable in an outer scope. In such cases, the inner variable shadows the outer variable, making the outer variable inaccessible within the inner scope.
Best Practices:
- Minimize the use of global variables and prefer passing values as function arguments.
- Use descriptive variable names to avoid Python scope-related confusion.
- Avoid variable shadowing to maintain code clarity and prevent unintended behavior.
- Be aware of scope-related issues when working with nested functions.
Understanding and effectively managing variable scope is crucial for writing clean, modular, and maintainable Python code. By following scope rules and adopting best practices, you can ensure proper variable usage and prevent scope-related bugs.
Here is some more detail on the code:
Local Scope: A variable defined within a function has a local scope. It is only accessible within that function. Once the function execution is completed, the local variable’s scope is destroyed, and the variable is no longer accessible.
def example_function():
local_variable = 10
print(local_variable) # Accessible within the functionexample_function()
print(local_variable) # Raises NameError, not accessible outside the function
Enclosing (Non-local) Scope: Variables defined in an enclosing function are accessible within nested functions. This scope allows inner functions to access variables from the outer function, but not vice versa.
def outer_function():
outer_variable = 5
def inner_function():
print(outer_variable) # Accessible within inner_function
Global Scope: Variables defined at the top level of a Python module or script have a global scope. They are accessible from any part of the module.
global_variable = 42 # Global scope
def example_function():
print(global_variable) # Accessible within the functionexample_function()
Built-in Scope: The built-in scope includes Python’s predefined functions and objects. These names are accessible globally.
print(len([1, 2, 3])) # len is a built-in function
When you reference a variable in your code, Python follows the LEGB rule for Python scope resolution, which stands for Local, enclosing, global, and built-in scopes. Python searches for a name in this order, starting with the local scope and progressing to higher scopes until it finds the variable or raises a NameError
if the name is not found.
Python variable scope inside if
In Python, an if
statement is a conditional statement that allows for the conditional execution of code based on a given condition. When if
statements are nested, variables defined within each if
block have different scopes. Here’s an explanation of the variable scope inside a if
statement:
- Variable Scope Inside an
if
Statement:- When a variable is defined inside an
if
the statement, its Python scope is limited to that particularif
block. - Variables defined within the
if
block are accessible only within that block and any nested blocks. - Once the execution exits the
if
block, the variables defined within it are no longer accessible.
- When a variable is defined inside an
Example:
x = 5 if x > 0: y = x * 2 print(y) # Output: 10 print(y) # Output: 10 if x < 0: z = x * 3 print(z) # This block will not execute since x is not less than 0 print(z) # Raises NameError: name 'z' is not defined
In the above example, the variable y
is defined within the first if
block and can be accessed within that block as well as outside it because it is defined in an outer scope. However, the variable z
is defined within the second if
block and is only accessible within that block. If we try to access z
outside of the if
block, a NameError
will be raised since z
is not defined in the outer scope.
It’s important to note that the Python scope of variables inside a if
statement is determined by the indentation level (block structure) rather than the if
condition itself. Proper indentation is crucial in Python to define the scope correctly and avoid unintended scoping issues.
In summary, variables defined inside an if
the statement has a scope limited to that specific if
block and any nested blocks. They are not accessible outside of the if
block or in other branches of the if
statement.
Python variable scope for loop
Variable scope in Python, a loop, such as a for
loop or a while
loop is a control structure that allows you to repeatedly execute a block of code. The variable scope inside a loop depends on where the variable is defined and how it is accessed within the loop. Here’s an explanation of the variable Python scope inside a loop:
- Variable Scope Inside a Loop:
- Variables defined before the loop or outside the loop have a broader scope and are accessible both inside and outside the loop.
- Variables defined within the loop have a local scope and are accessible only within the loop block.
Example 1: Variable defined outside the loop
x = 5 for i in range(3): print(x) # Output: 5 x += 1 print(x) # Output: 8
In the above example, the variable x
is defined before the for
loop, so it has a global scope. It can be accessed and modified both inside and outside the loop.
Example 2: Variable defined inside the loop
for i in range(3): y = i * 2 print(y) # Output: 0, 2, 4 print(y) # Raises NameError: name 'y' is not defined
In this example, the variable y
is defined within the for
loop. It has a local scope and can only be accessed within the loop block. Once the loop is exited, the variable y
is no longer accessible, and attempting to access it outside the loop will result in a NameError
.
It’s important to note that variables defined within a loop are re-initialized for each iteration of the loop. This means that the variable is effectively created anew with each iteration, even if it has the same name. If you need to access the value of a variable from a previous iteration outside the loop, you should define it before the loop.
In summary, variables defined outside a loop have a broader Python scope and can be accessed both inside and outside the loop. Variables defined inside a loop have a local scope and are only accessible within the loop block. Understanding variable scope inside loops is important for correctly accessing and managing variables in your code.
What are Python scope rules?
Python follows a set of scope rules to determine the accessibility and visibility of variables within different parts of the code. The scope rules in Python are as follows:
- Local Scope (Function Scope):
- Variables defined within a function are considered to have local scope.
- Local variables are accessible only within the function in which they are defined.
- Local variables are created when the function is called and destroyed when the function execution completes.
- Enclosing Scope (Nonlocal Scope):
- In nested functions, the enclosing scope refers to the Python scope of the immediate outer function.
- Variables defined in the enclosing scope can be accessed and modified within the inner function.
- However, modifications made to the variable in the inner function do not affect the value of the variable in the enclosing scope.
- Global Scope:
- Variables defined at the top level of a module or explicitly declared as global have global scope.
- Global variables are accessible from anywhere within the module.
- They can be accessed and modified for both inside and outside functions.
- Global variables are created when the module is imported or executed and exist until the execution of the program ends.
- Built-in Scope:
- The built-in scope refers to the pre-defined names and functions that are available in Python by default.
- It includes built-in functions like
print()
,len()
, and built-in types likeint
,str
, etc. - The built-in Python scope is accessible from anywhere in the code without the need for any import statements.
Python follows the “LEGB” rule to determine the order in which it searches for variables:
- Local scope (L): variables defined within the current function.
- Enclosing scope (E): Variables defined in the immediate outer function.
- Global scope (G): Variables defined at the top level of the module or explicitly declared as global.
- Built-in scope (B): Pre-defined names and functions provided by Python.
When a variable is accessed, Python starts the search from the local scope and moves up the scope hierarchy until it finds the variable. If the variable is not found in any of the Python scopes, a NameError
is raised.
Understanding the scope rules in Python helps in proper variable management, avoiding naming conflicts, and ensuring the correct accessibility and visibility of variables within the code.
See Also:
- Unveiling the Distinctions: Web Crawler vs Web Scrapers
- How to Make Money with Web Scraping Using Python
- How to Type Cast in Python with the Best 5 Examples
- Best Variable Arguments in Python
- 5 Best AI Prompt Engineering Certifications Free
- 5 Beginner Tips for Solving Python Coding Challenges
- Exploring Python Web Development Example Code
- “Python Coding Challenges: Exercises for Success”
- ChatGPT Prompt Engineering for Developers:
- How to Make AI-Generated Video|AI video generator
- 12 Best Python Web Scraping Tools and Libraries with Pros & Cons
- 7 Best Python Web Scraping Library To Master Data Extraction
- Explore the World of Character AI Generator
- Boolean Operators in Python Examples (And|Or|Not)
- Python List Slicing: 3 Best Advanced Techniques