How to Add Numpy Append Array | Empty Array Append | Column:
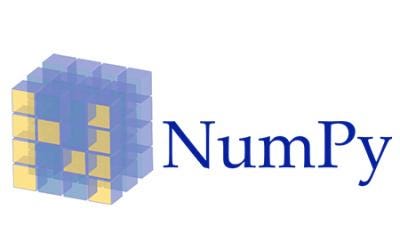
Using the numpy append() function in Python, two arrays can be combined. This function returns a new array; it does not modify the original array. In Python, the pre-defined method append is used to add a single item to specific types of collections. To add a single value or item, developers would need to modify the collection’s complete code if the append method didn’t exist. A list collection type is its main use.
The function concatenate() can also be used to add data to a numpy array. This function lets you concatenate along an existing axis; it takes as its first input a tuple or list of arrays. Arr1 and Arr2 have been concatenated in this example along the default axis (axis=0).
What does NumPy append do?
NumPy’s append
function is used to append values to the end of an array. It can be used to add elements to an existing NumPy array, creating a new array with the appended values. The syntax numpy.append
is as follows:
numpy.append(array, values, axis=None)
array
: The input array to which you want to append valuesvalues
: The values you want to append. This can be a scalar, an array-like object, or another NumPy array.axis
(optional): The axis along which the values will be appended. If not specified, the array is flattened before appending.
Here’s an example of how to use itnumpy.append
:
import numpy as np
# Create an initial NumPy array
arr = np.array([1, 2, 3])# Append a value to the end
new_arr = np.append(arr, 4)print(new_arr)
In this example, we append the value 4
to the end of the originalarr
, creating a new array new_arr
with the appended value.
You can also append multiple values by passing an array-like object as the values
argument:
import numpy as np
arr = np.array([1, 2, 3])
values_to_append = [4, 5, 6]new_arr = np.append(arr, values_to_append)
print(new_arr)
In this case, values_to_append
is appended to the end of arr
.
Keep in mind that numpy.append
creates a new array with the appended values and does not modify the original array. If you want to modify the original array in place, you should use other methods or perform the append operation directly on the original array.
How to add numpy append to array:
To append elements to a NumPy array, you can use NumPy functions to create a new array with the appended values. NumPy does not provide a built-in method to modify an array in place, as arrays in NumPy have a fixed size. Here’s how you can append elements to a NumPy array:
import numpy as np
# Create an initial NumPy array
arr = np.array([1, 2, 3])# Append a value to the end by creating a new array
value_to_append = 4
new_arr = np.append(arr, value_to_append)print(new_arr)
In this example, we create a new NumPy array, new_arr
, by appending the value 4
to the end of the original array arr
.
If you want to append multiple values, you can do so by passing an array-like object as the values
argument:
import numpy as np
arr = np.array([1, 2, 3])
values_to_append = [4, 5, 6]new_arr = np.append(arr, values_to_append)
print(new_arr)
This will create a new array, new_arr
, with the values [1, 2, 3, 4, 5, 6]
appended to the end.
Remember that NumPy’s append
function creates a new array with the appended values and does not modify the original array. If you want to modify the original array in place, you would need to use other NumPy functions or directly assign values to specific indices in the original array.
How to add a Numpy append to empty array:
If you want to add elements to an empty NumPy array, you can use the numpy.append
function just as you would with a non-empty array. Here’s how you can do it:
import numpy as np
# Create an empty NumPy array
empty_array = np.array([])# Define values to append
values_to_append = np.array([1, 2, 3, 4, 5])# Append the values to the empty array
result = np.append(empty_array, values_to_append)print(result)
In this example, we first create an empty NumPy array named empty_array
. Then, we define a separate NumPy array values_to_append
containing the values we want to add. We used np.append
to append the values values_to_append
to the empty array empty_array
. The result is a new array that contains the appended values.
Keep in mind that when appending to an empty array, the resulting array is a new array that includes the values from the original empty array as well as the values you’re appending. The original empty array remains unchanged.
How to append two NumPy Arrays?
numpy.concatenate
function, which allows you to concatenate arrays along a specified axis. Here’s how you can append two NumPy arrays together:
import numpy as np
# Create two NumPy arrays
array1 = np.array([1, 2, 3])
array2 = np.array([4, 5, 6])# Append array2 to array1 along axis 0 (vertical stacking)
result = np.concatenate((array1, array2), axis=0)print(result)
In this example, np.concatenate
is used to append array2
to the end of array1
along axis=0
, effectively performing vertical stacking. The result result
contains all elements from both arrays.
If you want to append the arrays horizontally (side by side), you can use axis=1
:
result = np.concatenate((array1, array2), axis=1)
array1
and array2
are 1D arrays, so appending them along either axis 0 or axis 1 is possible. If you’re working with 2D arrays or matrices, make sure the dimensions along the chosen axis match.How to Add a Numpy Append Column (With Examples)
In NumPy, you can append a column to a 2D array or matrix by using the numpy.c_
or numpy.column_stack
functions. Here’s how you can add a Numpy append column to an existing 2D array:
import numpy as np
# Create an initial 2D array (a matrix)
matrix = np.array([[1, 2, 3],
[4, 5, 6],
[7, 8, 9]])# Create a new column to append
new_column = np.array([10, 11, 12])# Append the new column to the matrix using numpy.c_
result = np.c_[matrix, new_column]print(result)
In this example, we create a new 2D array matrix
and a new column, and then we use np.c_
to append the new column to the existing matrix. The resultresult
will be the matrix with the new column added.
You can also achieve the same result using np.column_stack
:
result = np.column_stack((matrix, new_column))
Both methods perform the same operation and add a new column to the right side of the existing matrix. The original matrix remains unchanged, and a new matrix with the additional column is created.
Make sure that the shapes of the matrix and the new column are compatible and that they have the same number of rows, as you can’t append columns with different numbers of rows.
Numpy append vs Append column:
In NumPy, there might be some confusion surrounding the terms “numpy append” and “append column.” It’s important to clarify their meanings:
- NumPy
append
:numpy.append
is a function in NumPy used to append elements (values) to an existing array, whether it’s a 1D array or a 2D array (matrix).- You can use
numpy.append
to add values at the end of an array. It creates a new array with the appended values. - The function can be used for various appending scenarios, and it can be used for both 1D and 2D arrays. You can append values along a specified axis or flatten the array if the axis is not specified.
- Example:
np.append(array, values, axis=None)
- Append Column:
- “Append column” typically refers to the process of adding a new column to an existing 2D NumPy array or matrix. This can be done using functions like
np.c_
,np.column_stack
, or direct array manipulation to achieve the same result. - The term “append column” specifically focuses on adding an entire column to a 2D array. This operation usually implies adding a column of values to the right side of the existing matrix.
- “Append column” typically refers to the process of adding a new column to an existing 2D NumPy array or matrix. This can be done using functions like
Here’s an example of appending a column to a 2D NumPy array:
import numpy as np
# Create a 2D array (matrix)
matrix = np.array([[1, 2],
[3, 4],
[5, 6]])# Create a new column to append
new_column = np.array([7, 8, 9])# Append the new column to the matrix
result = np.c_[matrix, new_column]print(result)
In this example, we’re appending a new column, new_column
, to the right side of the existing matrix using np.c_
. This is an example of appending a column to a 2D NumPy array.
In summary, “numpy append” is a general operation for appending values to an array, while “append column” is a specific operation focused on adding columns to a 2D array or matrix. The latter is typically used when working with structured data or tabular data, where columns represent different variables or features.
Numpy append vs Concatenate:
NumPy provides two main functions for combining arrays: numpy.append
and numpy.concatenate
. While both can be used to combine arrays, they have some key differences in terms of usage and behavior:
numpy.append
:- Usage:
numpy.append(array, values, axis=None)
- Numpy appends value to an existing array.
- It can be used to append values to the end of an array (along a specified axis) or to flatten the array if the axis is not specified.
- Returns a new array with the appended values.
- If the axis is not specified, it flattens both the original and the appended arrays before concatenation.
- Typically used for simple appending of values, it can be less efficient than
numpy.concatenate
when used repeatedly in a loop because it creates a new array each time it’s called.
- Usage:
numpy.concatenate
:- Usage:
numpy.concatenate((arrays, axis=0, out=None))
- Combines multiple arrays along a specified axis.
- It’s more versatile and can concatenate multiple arrays along a specified axis. It can be used for vertical stacking (
axis=0
) or horizontal stacking (axis=1
) and can concatenate multiple arrays at once. - Returns a new concatenated array.
- Often more efficient when you need to concatenate multiple arrays because it doesn’t create unnecessary intermediate arrays.
- Suitable for more complex concatenation tasks, such as joining multiple arrays into a single, larger array.
- Usage:
Here’s a simple comparison between the two numpy append and concatenates:
import numpy as np
# Using numpy.append
arr1 = np.array([1, 2, 3])
arr2 = np.array([4, 5, 6])
result_append = np.append(arr1, arr2)
# result_append = [1, 2, 3, 4, 5, 6]# Using numpy.concatenate
result_concat = np.concatenate((arr1, arr2))
# result_concat = [1, 2, 3, 4, 5, 6]# Example of concatenating along the rows (vertical stacking)
matrix1 = np.array([[1, 2], [3, 4]])
matrix2 = np.array([[5, 6]])
result_concat_rows = np.concatenate((matrix1, matrix2), axis=0)
# result_concat_rows = [[1, 2], [3, 4], [5, 6]]# Example of concatenating along the columns (horizontal stacking)
matrix3 = np.array([[7], [8]])
result_concat_columns = np.concatenate((matrix1, matrix3), axis=1)
# result_concat_columns = [[1, 2, 7], [3, 4, 8]]
In summary, usenumpy.append
when you want to add values to the end of an array and use numpy.concatenate
when you need to combine multiple arrays along a specified axis, whether it’s for vertical or horizontal stacking.